How to Create a Chat App in Android - A Comprehensive Guide
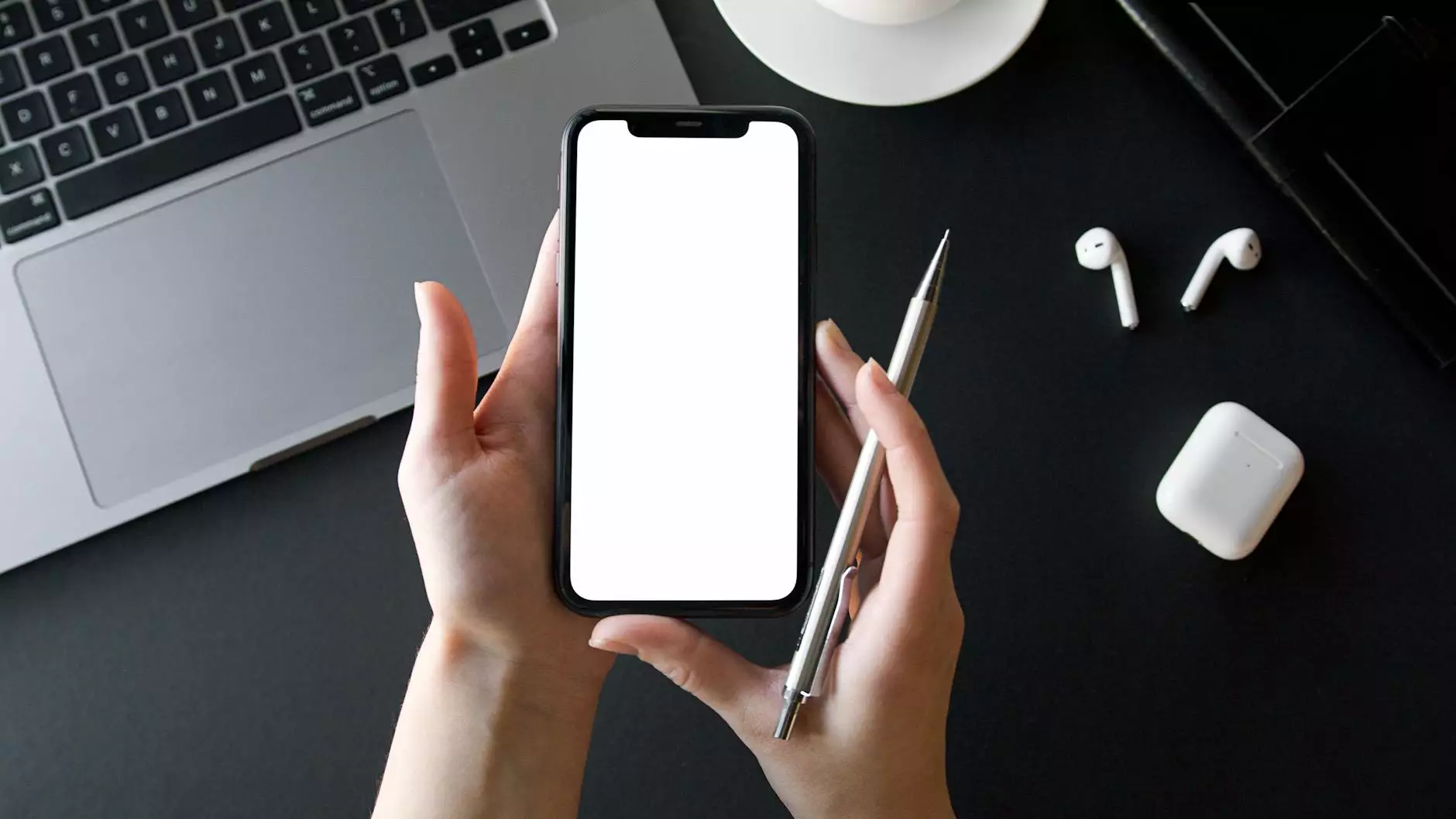
Creating a chat application for Android can seem like a daunting task, but with the right knowledge and tools, it’s absolutely achievable. This guide will take you through the entire process, from planning your app to deploying it on the Google Play Store. Whether you are a seasoned developer or a novice, by the end of this article, you will be equipped to build a functional chat app in Android. Let’s dive in!
1. Why Create a Chat App?
The popularity of messaging apps is on the rise. A chat application can serve various purposes, including:
- Instant Communication: Enable users to chat in real-time.
- Community Building: Foster connections through group chats.
- Business Use: Provide support and interaction with customers.
2. Planning Your Chat App
Before any coding begins, it’s crucial to spend some time planning your chat app. Consider the following aspects:
2.1 Define Your Target Audience
Understanding who your users are will guide many design and functionality decisions. Ask yourself:
- Are they teenagers, adults, or businesses?
- What features will they most likely need?
2.2 Key Features to Include
Essential functionalities might include:
- User registration and login
- Real-time messaging
- Push notifications for new messages
- User profiles and status updates
- Group chats
- Media sharing (images, videos, files)
3. Setting Up Your Development Environment
Once you have a plan, you can start setting up your development environment.
3.1 Required Tools and Frameworks
Here’s what you need to get started:
- Android Studio: The official IDE for Android development.
- Java or Kotlin: The programming languages you will use for app development.
- Firebase: A backend-as-a-service that provides real-time database capabilities.
3.2 Configure Android Studio
To set up your coding environment:
- Download and install Android Studio from the official website.
- Open Android Studio and start a new project using an Empty Activity.
- Choose either Java or Kotlin as your programming language.
4. Creating the User Interface
The user interface (UI) is essential in any chat application. It must be intuitive and user-friendly. Here’s how to get started:
4.1 Design the Layout
Using `XML`, design your layout files. Typical screens include:
- Login Screen
- Chat List Screen
- Individual Chat Screen
4.2 Implementing RecyclerView
To display chat messages, you can use RecyclerView. It provides an efficient way to show lists in Android:
- Create a new XML layout file for an individual chat item.
- Set up a RecyclerView in your chat screen's layout.
- Bind the RecyclerView to your data model using an adapter.
5. Building the Backend with Firebase
To support real-time communication, a robust backend service is essential. Firebase offers excellent support for chat applications.
5.1 Setting Up Firebase
To set up Firebase:
- Go to the Firebase Console and create a new project.
- Register your app within the project settings by providing the application package name.
- Download the configuration file (`google-services.json`) and place it in your app's `app/` directory.
5.2 Implementing Firebase Realtime Database
For real-time messaging, use Firebase’s Realtime Database:
- Define the database structure for user profiles and messages.
- Use Firebase SDK to read and write messages from your app.
- Set up listeners to update the UI when new messages arrive.
6. Adding User Authentication
To allow users to safely communicate via your app, implement user authentication. Firebase provides easy user management.
6.1 Using Firebase Authentication
- Enable authentication methods (Google, Email/Password) in the Firebase Console.
- Implement login and registration screens in your app.
- Use Firebase Authentication APIs to manage user sessions.
7. Implementing Real-Time Messaging
The heart of any chat app is its messaging functionality. Here’s how to implement it:
7.1 Sending Messages
To send messages:
- Create a message model to structure your data (sender, receiver, message text, timestamp).
- Use Firebase to push new messages to the database when a user sends a message.
7.2 Receiving Messages
To receive messages, set up listeners on the appropriate database nodes. Every time a new message arrives, update your RecyclerView so users can see it.
8. Integrating Push Notifications
To enhance user experience, integrate push notifications. This will alert users when they receive new messages, even when they aren’t using the app.
8.1 Setting Up Firebase Cloud Messaging
- Enable Cloud Messaging in your Firebase Console.
- Integrate the necessary dependencies into your Android project.
- Handle notification messages within your app to display notifications correctly.
9. Testing Your Application
Before launching your app, it is crucial to perform comprehensive tests. Consider:
- Unit Testing: Check individual components of your chat app.
- Integration Testing: Ensure that different components work together seamlessly.
- User Acceptance Testing: Gather feedback from potential users to refine the app.
10. Launching Your Chat App
Once everything is tested and working correctly, it’s time to launch your app on the Google Play Store:
- Prepare marketing materials (screenshots, app description, icons).
- Follow the guidelines for app submission and complete the submission process.
- Monitor user feedback and be ready to implement updates and improvements based on real-world use.
Conclusion
Creating a chat app in Android may seem complex, but by breaking it down into manageable components, anyone can do it. With the power of tools like Firebase and Android Studio, you can develop a feature-rich application that provides real-time communication. By following this detailed guide on how to create a chat app in Android, you are well on your way to building an engaging and user-friendly chat platform. Embrace the journey and happy coding!